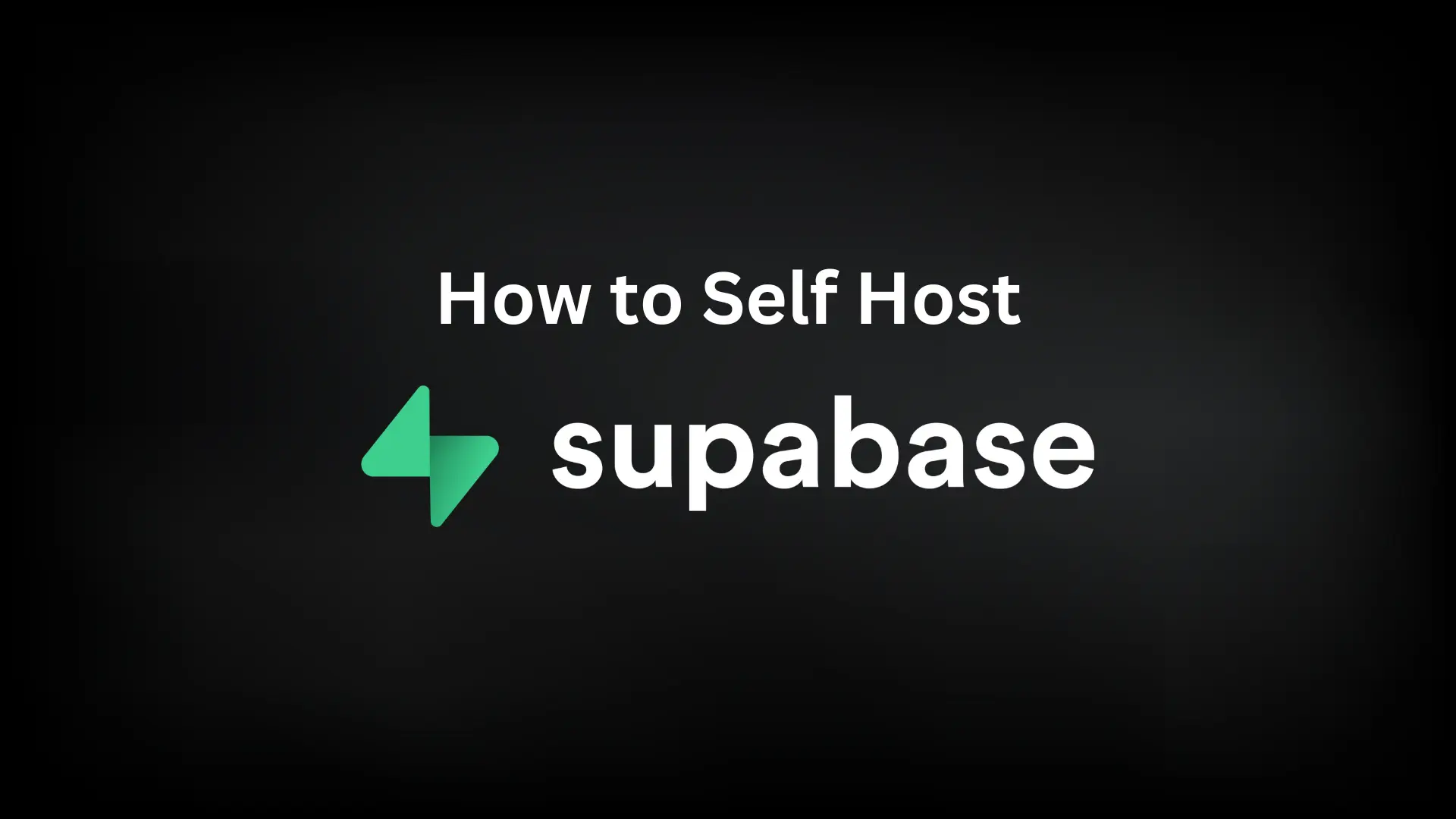
How to Self-Host Supabase: A Practical Guide
April 7, 2025
Learn how to self-host Supabase for complete control over your data, enhanced privacy, and potential cost savings with this step-by-step guide.
Fernando Chaves
August 4th, 2024
Discover how to seamlessly integrate Supabase with React Native and Expo, empowering your mobile applications with a robust, scalable backend. This comprehensive guide walks you through the process, showcasing Supabase React Native examples and exploring its features and pricing.
Before we dive into the integration process, let's briefly discuss why Supabase is an excellent choice for React Native developers:
Supabase offers a range of pricing options to suit different project needs:
For most React Native projects, the Free or Pro plan will suffice. You can upgrade as your app scales.
Let's start by creating a fresh Expo project:
npx create-expo-app my-supabase-react-native-app
cd my-supabase-react-native-app
Install the Supabase JavaScript client library:
npx expo install @supabase/supabase-js
Create a new project in your Supabase dashboard. Once created, navigate to the project settings to find your API URL and public API key.
Create a new file named `supabase.js` in your project root:
import { createClient } from '@supabase/supabase-js';
const supabaseUrl = 'YOUR_SUPABASE_PROJECT_URL';
const supabaseKey = 'YOUR_SUPABASE_PUBLIC_API_KEY';
export const supabase = createClient(supabaseUrl, supabaseKey);
Replace 'YOUR_SUPABASE_PROJECT_URL' and 'YOUR_SUPABASE_PUBLIC_API_KEY' with your actual Supabase project details.
Here's a practical example of using Supabase in your React Native components to fetch data:
import React, { useEffect, useState } from 'react';
import { View, Text, FlatList, StyleSheet } from 'react-native';
import { supabase } from './supabase';
export default function UserList() {
const [users, setUsers] = useState([]);
const [loading, setLoading] = useState(true);
useEffect(() => {
fetchUsers();
}, []);
async function fetchUsers() {
try {
setLoading(true);
const { data, error } = await supabase
.from('users')
.select('*')
.limit(10);
if (error) throw error;
setUsers(data);
} catch (error) {
console.error('Error fetching users:', error.message);
} finally {
setLoading(false);
}
}
return (
<View style={styles.container}>
<Text style={styles.title}>User List</Text>
{loading ? (
<Text>Loading users...</Text>
) : (
<FlatList
data={users}
keyExtractor={(item) => item.id.toString()}
renderItem={({ item }) => (
<Text style={styles.userItem}>{item.name}</Text>
)}
/>
)}
</View>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
padding: 20,
backgroundColor: '#f0f0f0',
},
title: {
fontSize: 24,
fontWeight: 'bold',
marginBottom: 20,
},
userItem: {
fontSize: 16,
marginBottom: 10,
padding: 10,
backgroundColor: '#ffffff',
borderRadius: 5,
},
});
Supabase provides built-in authentication. Here's how to implement a simple sign-up function in your React Native app:
import { supabase } from './supabase';
async function signUp(email, password) {
try {
const { user, error } = await supabase.auth.signUp({
email: email,
password: password,
});
if (error) throw error;
console.log('User signed up successfully:', user);
return user;
} catch (error) {
console.error('Error signing up:', error.message);
return null;
}
}
Supabase offers several advanced features that can enhance your React Native app:
Integrating Supabase with React Native and Expo opens up a world of possibilities for building powerful, scalable mobile applications. With its generous free tier and straightforward pricing structure, Supabase is an excellent choice for developers of all levels. As you continue to explore Supabase's features, you'll find it to be a robust and cost-effective solution for your backend needs.
By mastering the integration of Supabase with React Native and Expo, you're well-equipped to create sophisticated, data-driven mobile applications that can scale with your needs. Happy coding!
April 7, 2025
Learn how to self-host Supabase for complete control over your data, enhanced privacy, and potential cost savings with this step-by-step guide.
December 9, 2024
Learn how to set up Push Notifications in React Native using Expo Notifications and Firebase Cloud Messaging (FCM) for effective user engagement.
July 22, 2024
Learn how to set up Expo with NativeWind for efficient styling in your React Native projects.