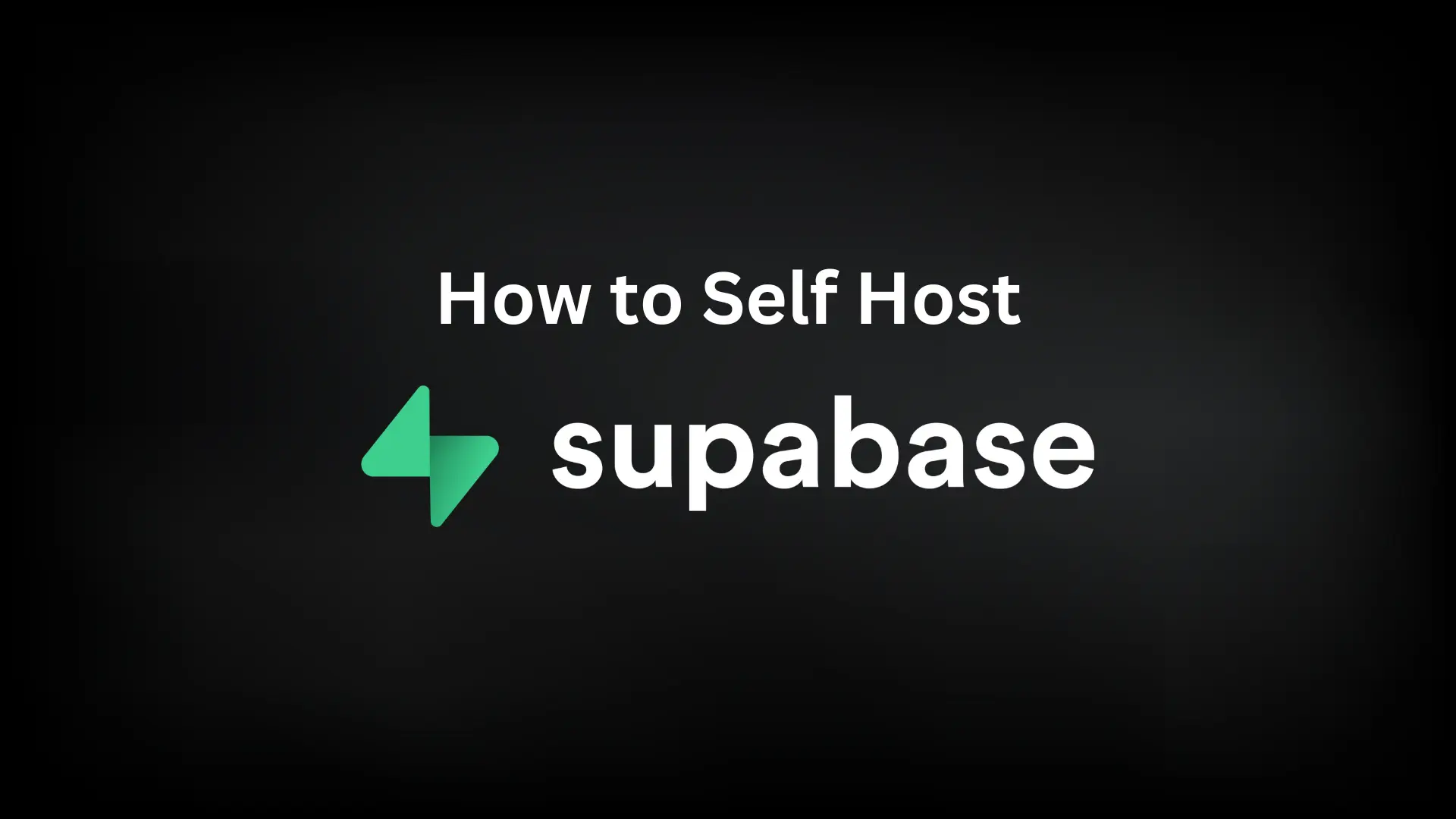
How to Self-Host Supabase: A Practical Guide
April 7, 2025
Learn how to self-host Supabase for complete control over your data, enhanced privacy, and potential cost savings with this step-by-step guide.
Fernando Chaves
October 4th, 2024
In the ever-evolving landscape of React development, choosing the right state management library can make or break your project. As we step into 2024, two contenders stand out in the ring: the battle-tested Redux and the rising star Zustand. But which one should you choose for your next React application? Let's dive deep into the world of state management and explore the strengths, weaknesses, and use cases of Redux and Zustand.
Before we pit Redux against Zustand, it's crucial to understand why state management libraries are essential in React applications. As your app grows in complexity, managing state across components becomes challenging. Props drilling, unnecessary re-renders, and convoluted data flow can quickly turn your codebase into a tangled mess. This is where state management libraries come to the rescue, offering structured approaches to handle shared state and keep your app organized and performant.
Redux has long been the go-to solution for state management in React applications. Introduced in 2015, Redux follows the Flux architecture and provides a predictable state container for JavaScript apps. Let's explore what makes Redux a popular choice among developers.
// Example of Redux setup
import { createStore } from 'redux';
// Reducer
const counterReducer = (state = { count: 0 }, action) => {
switch (action.type) {
case 'INCREMENT':
return { count: state.count + 1 };
case 'DECREMENT':
return { count: state.count - 1 };
default:
return state;
}
};
// Store
const store = createStore(counterReducer);
// Action creators
const increment = () => ({ type: 'INCREMENT' });
const decrement = () => ({ type: 'DECREMENT' });
// Usage
store.dispatch(increment());
console.log(store.getState()); // { count: 1 }
Enter Zustand, a relatively new player in the state management arena. Created by the developers behind Jotai and React-spring, Zustand offers a minimalist approach to state management. Let's explore what makes Zustand an attractive alternative to Redux.
// Example of Zustand setup
import create from 'zustand';
const useStore = create((set) => ({
count: 0,
increment: () => set((state) => ({ count: state.count + 1 })),
decrement: () => set((state) => ({ count: state.count - 1 })),
}));
// Usage in a React component
function Counter() {
const { count, increment, decrement } = useStore();
return (
<div>
<p>Count: {count}</p>
<button onClick={increment}>Increment</button>
<button onClick={decrement}>Decrement</button>
</div>
);
}
Now that we've explored the features of both Redux and Zustand, let's compare them directly on various aspects to help you make an informed decision for your React project.
Redux has a steeper learning curve due to its concepts like actions, reducers, and middleware. It requires understanding the Flux architecture and immutability principles. On the other hand, Zustand's simplicity and hook-based approach make it much easier to learn and implement, especially for developers already familiar with React hooks.
Redux is known for requiring a significant amount of boilerplate code to set up stores, define actions, and create reducers. This can be time-consuming and may feel overwhelming for smaller projects. Zustand, with its minimal API, requires much less setup and boilerplate, allowing developers to get started quickly.
In terms of performance, Zustand often has an edge, especially in smaller to medium-sized applications. Its lightweight nature and efficient update mechanism can lead to better performance. Redux, while generally performant, can introduce some overhead due to its centralized store and the way it handles updates.
Redux shines in large, complex applications where its structured approach to state management helps maintain order and predictability as the app grows. Zustand, while flexible, may require more discipline to keep the state management organized in very large projects. However, for small to medium-sized applications, Zustand's simplicity can lead to more maintainable code.
Redux boasts a large, mature ecosystem with numerous middleware, extensions, and tools available. It has been battle-tested in production for years and has extensive community support. Zustand, being newer, has a smaller but growing ecosystem. While it may not have as many third-party tools, its simplicity often means you need fewer additional libraries.
Redux offers powerful debugging capabilities through the Redux DevTools, which allow time-travel debugging and detailed inspection of state changes. Zustand provides basic debugging features and can be used with the Redux DevTools with some configuration, but the experience is not as comprehensive as native Redux debugging.
Choosing between Redux and Zustand ultimately depends on your project's specific needs, your team's expertise, and your development priorities. Here are some guidelines to help you decide:
As we look towards the future of React development in 2024 and beyond, both Redux and Zustand have their place in the ecosystem. Redux continues to be a robust solution for complex applications, while Zustand offers a fresh, lightweight approach that aligns well with modern React practices.
The trend towards simpler, more intuitive state management solutions is clear, with libraries like Zustand gaining popularity. However, Redux's maturity and extensive ecosystem ensure its continued relevance, especially in enterprise-level applications.
Ultimately, the best choice depends on your specific use case. By understanding the strengths and weaknesses of both Redux and Zustand, you can make an informed decision that will set your React project up for success. Remember, the goal is to choose a solution that enhances your development experience and helps you build efficient, maintainable React applications.
To dive deeper into Redux and Zustand, check out these official resources:
Whether you choose Redux, Zustand, or another state management solution, the key is to stay adaptable and open to learning. The React ecosystem is constantly evolving, and what works best today might change tomorrow. Keep exploring, experimenting, and sharing your experiences with the community to contribute to the ongoing evolution of React state management.
April 7, 2025
Learn how to self-host Supabase for complete control over your data, enhanced privacy, and potential cost savings with this step-by-step guide.
April 7, 2025
Learn how to secure your OpenAI, Anthropic, and other AI API keys from unauthorized access to prevent billing surprises and data breaches.
December 9, 2024
Learn how to set up Push Notifications in React Native using Expo Notifications and Firebase Cloud Messaging (FCM) for effective user engagement.