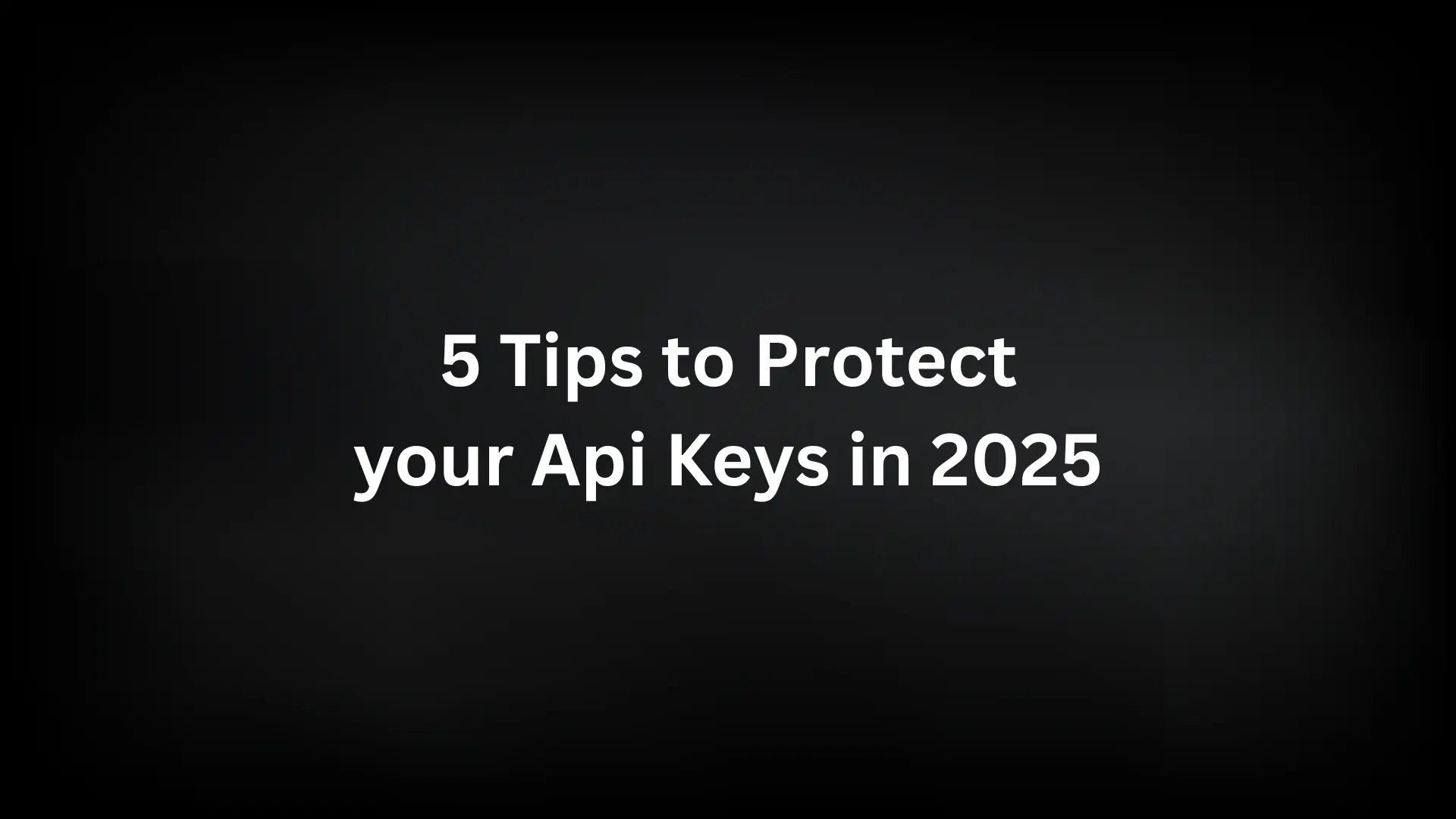
5 Tips to Protect Your AI API Keys in 2025
April 7, 2025
Learn how to secure your OpenAI, Anthropic, and other AI API keys from unauthorized access to prevent billing surprises and data breaches.
Fernando Chaves
October 2st, 2024
In the world of mobile app development, efficiently handling network requests is crucial for creating responsive and reliable applications. React Native, a popular framework for building cross-platform mobile apps, offers various tools and techniques for debugging network requests. In this comprehensive guide, we'll explore the best methods to debug network requests in React Native, helping you optimize your app's performance and quickly resolve API-related issues.
Before diving into the techniques, let's understand why debugging network requests is essential:
One of the most straightforward methods to debug network requests in React Native is using Chrome Developer Tools:
This method allows you to inspect request and response headers, bodies, and timing information. It's particularly useful for quick debugging sessions and doesn't require any additional setup.
For a more customized approach, you can implement console logging in your network request functions:
const fetchData = async () => {
try {
console.log('Fetching data...');
const response = await fetch('https://api.example.com/data');
console.log('Response status:', response.status);
const data = await response.json();
console.log('Received data:', data);
// Process data...
} catch (error) {
console.error('Error fetching data:', error);
}
};
This method allows you to log specific information at different stages of the network request, giving you more control over what you want to debug.
If you're using Expo for your React Native development, you can take advantage of the built-in Network Inspector:
The Expo Network Inspector provides a user-friendly interface for debugging network requests directly within your development environment.
For more advanced debugging capabilities, consider using third-party tools like Proxyman:
Tools like Proxyman offer powerful features for in-depth network debugging, making them ideal for complex debugging scenarios.
To make your debugging process more effective, implement proper error handling and retry logic in your network requests:
const fetchDataWithRetry = async (retries = 3) => {
for (let i = 0; i < retries; i++) {
try {
const response = await fetch('https://api.example.com/data');
if (!response.ok) throw new Error('Network response was not ok');
return await response.json();
} catch (error) {
console.error(`Attempt ${i + 1} failed: ${error.message}`);
if (i === retries - 1) throw error;
}
}
};
This approach helps you identify persistent network issues and distinguish between temporary failures and more serious problems.
Debugging network requests in React Native is a crucial skill for developing robust and efficient mobile applications. By utilizing the methods and tools discussed in this guide, you can effectively identify and resolve network-related issues, leading to improved app performance and user experience.
Remember, the key to successful debugging is a combination of the right tools, proper implementation of error handling, and a systematic approach to troubleshooting. As you become more proficient in these techniques, you'll be able to create more reliable and performant React Native applications.
While mastering debugging techniques is essential, starting with a solid foundation can significantly speed up your development process. LaunchYourApp offers a comprehensive React Native boilerplate that includes pre-configured networking modules, error handling, and debugging tools. Our solution allows you to focus on building your app's unique features while ensuring robust network handling out of the box.
Explore LaunchYourApp's FeaturesApril 7, 2025
Learn how to secure your OpenAI, Anthropic, and other AI API keys from unauthorized access to prevent billing surprises and data breaches.
December 9, 2024
Learn how to set up Push Notifications in React Native using Expo Notifications and Firebase Cloud Messaging (FCM) for effective user engagement.
October 14, 2024
An in-depth comparison of React Native and Ionic frameworks for mobile app development, helping you make the right choice for your project in 2024.